img2vid 
Generate videos or gifs from images. Includes captions, audio, transitions, zoompan and a watermark image.
Requires
ffmpeg with --enable-libass
Install
npm install img2vid
Example Usage
var img2vid = require('img2vid')
var payload = {
slides: [
{
path: 'examples/exampleData/squirrel.jpg',
duration: 2
},
{
path: 'examples/exampleData/cassettes.jpg',
duration: 2
},
{
path: 'examples/exampleData/mouse.jpg',
duration: 2
}
],
captions: [
{
text: 'Img2Vid',
start: 0,
end: 6
}
],
width: 640,
height: 419,
output: 'examples/outputs/simple.webp',
assOutput: 'examples/outputs/simple.ass',
forceScale: true
}
img2vid.render(payload)
Options
key |
required |
default |
type |
description |
slides |
Y |
|
[Object] |
See Slide Options |
captions |
N |
|
[Object] |
See Caption Options |
width |
Y |
640 |
Number |
Pixels |
height |
Y |
480 |
Number |
Pixels |
output |
Y |
output.mp4 |
String |
filepath.[mp4/gif/webp] |
assOutput |
Y iff using captions |
subs.ass |
String |
ASS File Output - filepath.ass |
audio |
N |
|
String |
Path to audio file |
genpalette |
N |
false |
Boolean |
Generate color palette for Gifs/Webp outputs |
forceScale |
N |
false |
Boolean |
Use if input images are different sizes |
duration |
N |
|
Number |
Output Duration |
gifLoop |
N |
true |
Boolean |
Continually loop Gifs/Webp outputs |
hardSub |
N |
true |
Boolean |
Render captions over output |
verbose |
N |
true |
Boolean |
Show Logs |
watermark |
N |
|
Object |
See Watermark Options |
Slide Options
Transition Options
ZoomPan Options
key |
required |
default |
type |
description |
startTime |
N |
0 |
Number |
Seconds from start of slide |
endTime |
Y |
|
Number |
Seconds to finish ZP |
zoomStart |
N |
1 |
Number |
Scale Factor to Start |
zoomEnd |
Y |
1 |
Number |
Scale Factor to End |
xStart |
N |
0 |
Number |
Start X Position |
yStart |
N |
0 |
Number |
Start Y Position |
xEnd |
Y |
0 |
Number |
End X Position |
yEnd |
Y |
0 |
Number |
End Y Position |
jitterScale |
N |
2 |
Number|False |
Temp scale image up to reduce jitter |
Caption Options
key |
required |
default |
type |
description |
text |
Y |
|
String |
image filepath |
start |
Y |
0 |
Number |
Seconds in total output |
end |
Y |
0 |
Number |
Seconds in total output |
style |
N |
|
Object |
See Style Options |
Caption Style Options
key |
alias |
default |
type |
description |
fontName |
fn |
Arial |
String |
[x,y] position in pixels |
fontSize |
fs |
28 |
Number |
|
borderSize |
bord |
2 |
Number |
|
color |
|
#FFFFFF |
String |
Must be 6 char hex color |
borderColor |
|
#000000 |
String |
Must be 6 char hex color |
fade |
fad |
|
[Number,Number] |
[fadeIn,fadeOut] in ms |
position |
pos |
|
[Number,Number] |
[x,y] in pixels |
lineAlignment |
an |
top left |
String |
top,middle,bottom left,center,right |
scaleX |
fscx |
100 |
Number |
as a percentage |
scaleY |
fscy |
100 |
Number |
as a percentage |
animate |
t |
|
String |
See animate string syntax |
move |
|
|
Number[4] |
[startX,startY,endX,endY] |
shadow |
shad |
|
Number |
depth of shadow |
shadowColor |
|
|
String |
Must be 6 char hex color |
shadowAlpha |
|
|
String |
Must be 2 char hex alpha color |
bold |
b |
1 |
Number |
1 or 0 |
italics |
i |
0 |
Number |
1 or 0 |
underline |
u |
0 |
Number |
1 or 0 |
strikeout |
s |
0 |
Number |
1 or 0 |
Watermark Options
key |
required |
default |
type |
description |
path |
Y |
0 |
String |
Path to watermark image |
height |
N |
100 |
Number |
Pixels (-1 to keep aspect ratio) |
width |
N |
-1 |
Number |
Pixels (-1 to keep aspect ratio) |
x |
N |
10 |
Number |
Pixels |
y |
N |
10 |
Number |
Pixels |
alpha |
N |
1 |
Number |
Opacity of image (Between 0 and 1) |
Available Slide Transitions
View transitions types and demos available from FFMPEG xfade filter.
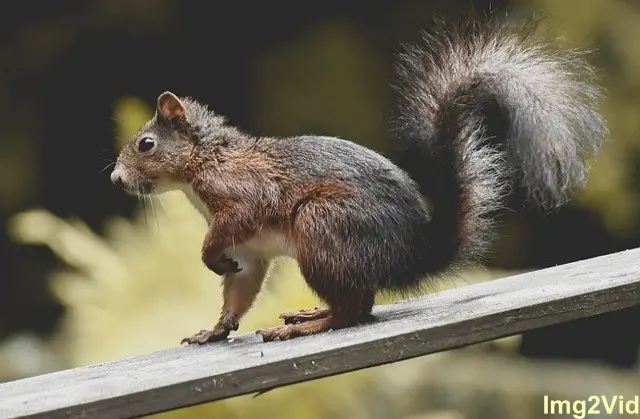