
Anymotion provides one unified API for animating UIKit, CoreAnimation, POP and your library of choice
- powerful oneliners
- grouping and chaining animations
- cancellable animations with callbacks for clean up
- swift api
Installation
Via CocoaPods
If you're using CocoaPods, you can simply add to your `Podfile`:
```ruby
pod 'Anymotion', :git => 'https://github.com/agens-no/Anymotion.git', :branch => 'master'
```
This will download the `Anymotion` and dependencies in `Pods/` during your next `pod install` exection. You may have to say `pod repo update` first.
##### Import in swift
```swift
import Anymotion
```
##### Import in Objective-C
```objc
#import
```
Via Carthage
To install via [Carthage](https://github.com/Carthage/Carthage) add to your Cartfile:
```ruby
github "agens-no/anymotion"
```
##### Import in swift
```swift
import Anymotion
```
##### Import in Objective-C
```objc
#import
```
Basics
Using a chainable builder pattern we can pack a good deal of configuration in one line
let goRight = ANYPOPSpring(kPOPLayerPositionX).toValue(100).springSpeed(5).animation(for: view.layer)
let fadeOut = ANYCABasic(#keyPath(CALayer.opacity)).toValue(0).duration(1).animation(for: view.layer)
Note: These animations won't start unless you say start
like this
goRight.start()
fadeOut.start() |
|
Instead of starting each one individually you can group them
goRight.groupWith(fadeOut).start() |
|
Calling start
actually returns an ANYActivity
empowering you to stop the animation at any time.
let activity = goRight.groupWith(fadeOut).start()
...
activity.cancel() |
|
Live Examples
Compile and run the iOS-Example project to watch some beautiful examples!
Frameworks integrations
POP
let spring = ANYPOPSpring(kPOPLayerPositionX)
.toValue(100)
.springSpeed(5)
.animation(for: view.layer)
|
|
let basic = ANYPOPBasic(kPOPLayerPositionX)
.toValue(100)
.duration(2)
.animation(for: view.layer)
|
|
let decay = ANYPOPDecay(kPOPLayerPositionX)
.velocity(10)
.animation(for: view.layer)
|
|
Core Animation
let basic = ANYCABasic(#keyPath(CALayer.position))
.toValue(CGPoint(x: 100, y: 0))
.duration(2)
.animation(for: view.layer)
|
|
UIKit
let uikit = ANYUIView.animation(duration: 2) {
view.center.x = 100
} |
|
Operators
Grouping
Start animations simultaneously
ANYAnimation *goRight = ...;
ANYAnimation *fadeOut = ...;
ANYAnimation *group = [ANYAnimation group:@[goRight, fadeOut]];
[group start]; |
|
Chaining
When one animation completes then start another
ANYAnimation *goRight = ...;
ANYAnimation *goLeft = ...;
ANYAnimation *group = [goRight then:goLeft];
[group start]; |
|
Repeat
ANYAnimation *goRight = ...;
ANYAnimation *goLeft = ...;
ANYAnimation *group = [[goRight then:goLeft] repeat];
[group start]; |
|
Set up and clean up
ANYAnimation *pulsatingDot = ...;
[[pulsatingDot before:^{
view.hidden = NO;
}] after:^{
view.hidden = YES;
}];
[pulsatingDot start]; |
|
Callbacks
ANYAnimation *anim = ...;
[[anim onCompletion:^{
NSLog(@"Animation completed");
} onError:^{
NSLog(@"Animation was cancelled");
}] start]; |
|
Feedback
We would 😍 to hear your opinion about this library. Wether you like it or don't. Please file an issue if there's something you would like like improved, so we can fix it!
If you use Anymotion and are happy with it consider sending out a tweet mentioning @agens. This library is made with love by Mats Hauge, who's passionate about animations, and Håvard Fossli, who cares deeply about architecture.
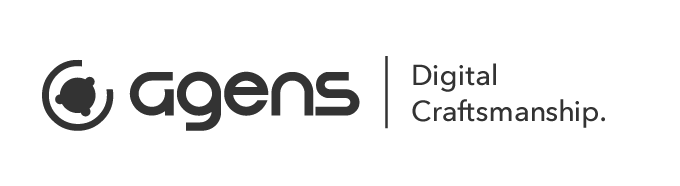