Closed rh101 closed 1 year ago
Do you check premultiplyAlpha when convert png to .pvr?
Do you check premultiplyAlpha when convert png to .pvr?
Good catch! The Texture Packer CLI script that processes all the graphics has ClearTransparentPixels
set for transparency handling, but changing it over to PremultiplyAlpha
fixed the issue! That script was created years ago, and I've never noticed this specific problem before from it, which is why I didn't even think to check it.
Thank you very much!
Using a simple program drawing a semi-transparent
Glow.png
image withSprite::create()
over a solid LayerColor or solid sprite, this should be the output:Image A (correct output - note the solid black under the glow)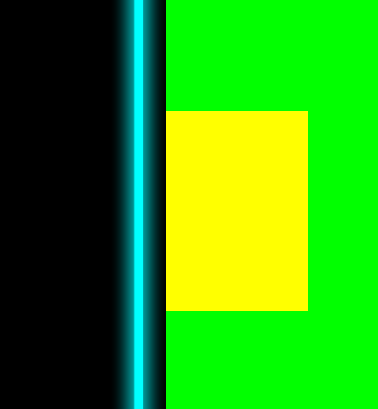
If I use PVR format for the file (such as
pvr.gz
orpvr.ccz
sprite sheet), and load withSprite::createWithFrameName()
, this is what happens:Image B (incorrect output - note that you can see the yellow box and background under the glow)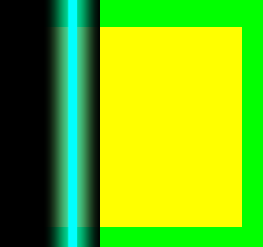
For some reason, the semi-transparent glow alpha section is blending in with the solid black and making it transparent, when it should be more like image A above.
Has anyone experienced this with PVR files? Using standalone PNG or PNG sprite sheets is perfectly fine, but PVR files are causing a problem. The PVR sprite sheet output was created with TexturePacker with the following settings:
At the moment I have absolutely no idea why it would be blending it in such a way. The code is just this (modifications to template cpp project):