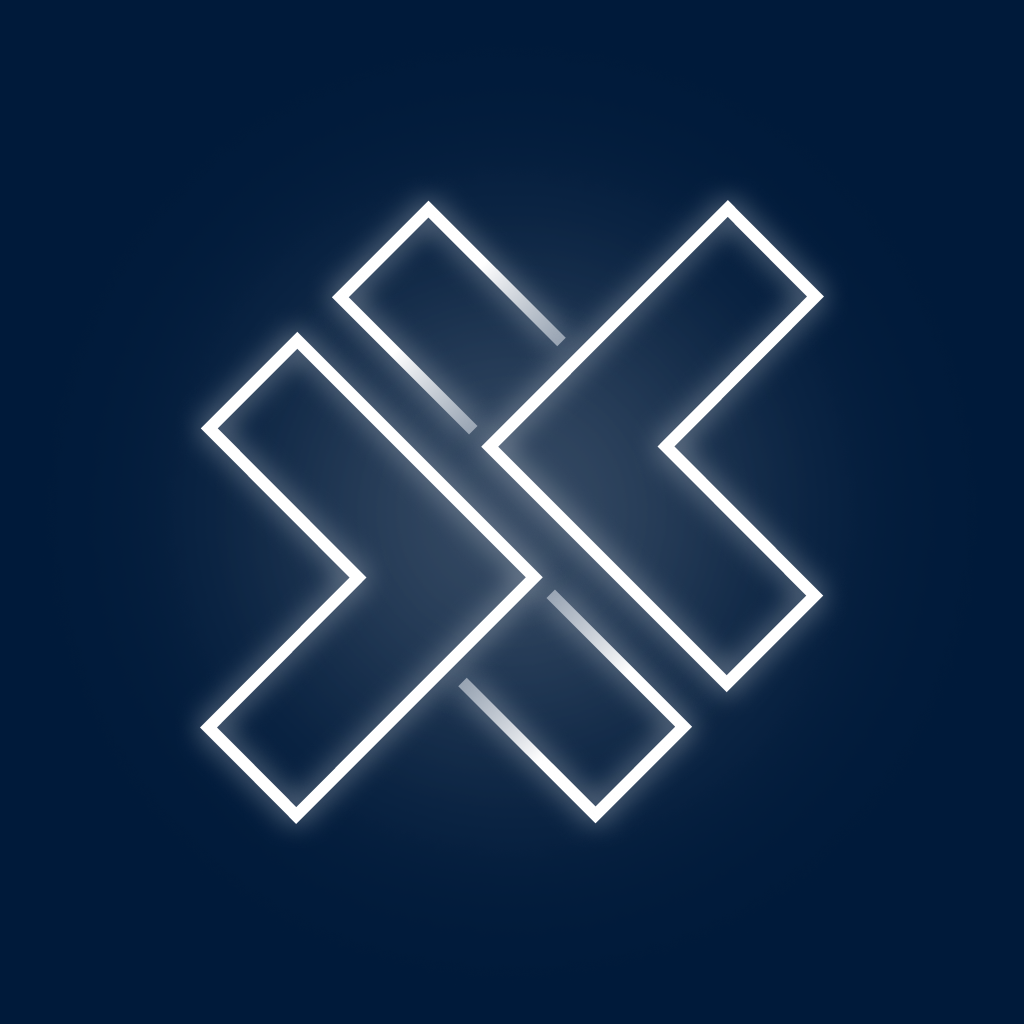
Capacitor Camera Preview
@capacitor-community/camera-preview
CAPACITOR 5
Capacitor plugin that allows camera interaction from Javascript and HTML
(based on cordova-plugin-camera-preview).
Version 6 of this plugin requires Capacitor 6.
If you are using Capacitor 5, use [version 5](https://github.com/capacitor-community/camera-preview/releases/tag/v5.0.0)
If you are using Capacitor 4, use [version 4](https://github.com/capacitor-community/camera-preview/releases/tag/v4.0.0)
If you are using Capacitor 3, use [version 3](https://github.com/capacitor-community/camera-preview/releases/tag/v3.1.2)
If you are using Capacitor 2, use [version 1](https://github.com/capacitor-community/camera-preview/releases/tag/v1.2.1)
**PR's are greatly appreciated.**
-- [@arielhernandezmusa](https://github.com/arielhernandezmusa) and [@pbowyer](https://github.com/pbowyer), current maintainers
# Installation
```
yarn add @capacitor-community/camera-preview
or
npm install @capacitor-community/camera-preview
```
Then run
```
npx cap sync
```
## Extra Android installation steps
**Important** `camera-preview` 3+ requires Gradle 7. If you are using Gradle 4, please use [version 2](https://github.com/capacitor-community/camera-preview/tree/v2.1.0) of this plugin.
Open `android/app/src/main/AndroidManifest.xml` and above the closing `` tag add this line to request the CAMERA permission:
```xml
```
For more help consult the [Capacitor docs](https://capacitorjs.com/docs/android/configuration#configuring-androidmanifestxml).
### Variables
This plugin will use the following project variables (defined in your app's `variables.gradle` file):
- `androidxExifInterfaceVersion`: version of `androidx.exifinterface:exifinterface` (default: `1.3.6`)
## Extra iOS installation steps
You will need to add two permissions to `Info.plist`. Follow the [Capacitor docs](https://capacitorjs.com/docs/ios/configuration#configuring-infoplist) and add permissions with the raw keys `NSCameraUsageDescription` and `NSMicrophoneUsageDescription`. `NSMicrophoneUsageDescription` is only required, if audio will be used. Otherwise set the `disableAudio` option to `true`, which also disables the microphone permission request.
## Extra Web installation steps
Add `import { CameraPreview } from '@capacitor-community/camera-preview';` in the file where you want to use the plugin.
then in html add `
`
and `CameraPreview.start({ parent: "cameraPreview"});` will work.
# Methods
### start(options)
Starts the camera preview instance.
| Option | values | descriptions |
|----------|---------------|------------------------------------------------------------------------|
| position | front \| rear | Show front or rear camera when start the preview. Defaults to front |
| width | number | (optional) The preview width in pixels, default window.screen.width (applicable to the android and ios platforms only) |
| height | number | (optional) The preview height in pixels, default window.screen.height (applicable to the android and ios platforms only) |
| x | number | (optional) The x origin, default 0 (applicable to the android and ios platforms only) |
| y | number | (optional) The y origin, default 0 (applicable to the android and ios platforms only) |
| toBack | boolean | (optional) Brings your html in front of your preview, default false (applicable to the android and ios platforms only) |
| paddingBottom | number | (optional) The preview bottom padding in pixes. Useful to keep the appropriate preview sizes when orientation changes (applicable to the android and ios platforms only) |
| rotateWhenOrientationChanged | boolean | (optional) Rotate preview when orientation changes (applicable to the ios platforms only; default value is true) |
| storeToFile | boolean | (optional) Capture images to a file and return back the file path instead of returning base64 encoded data, default false. |
| disableExifHeaderStripping | boolean | (optional) Disable automatic rotation of the image, and let the browser deal with it, default true (applicable to the android and ios platforms only) |
| enableHighResolution | boolean | (optional) Defaults to false - iOS only - Activate high resolution image capture so that output images are from the highest resolution possible on the device |
| disableAudio | boolean | (optional) Disables audio stream to prevent permission requests, default false. (applicable to web and iOS only) |
| lockAndroidOrientation | boolean | (optional) Locks device orientation when camera is showing, default false. (applicable to Android only) |
| enableOpacity | boolean | (optional) Make the camera preview see-through. Ideal for augmented reality uses. Default false (applicable to Android and web only)
| enableZoom | boolean | (optional) Set if you can pinch to zoom. Default false (applicable to the android and ios platforms only)
```javascript
import { CameraPreview, CameraPreviewOptions } from '@capacitor-community/camera-preview';
const cameraPreviewOptions: CameraPreviewOptions = {
position: 'rear',
height: 1920,
width: 1080
};
CameraPreview.start(cameraPreviewOptions);
```
Remember to add the style below on your app's HTML or body element:
```css
ion-content {
--background: transparent;
}
```
Take into account that this will make transparent all ion-content on application, if you want to show camera preview only in one page, just add a custom class to your ion-content and make it transparent:
```css
.my-custom-camera-preview-content {
--background: transparent;
}
```
If the camera preview is not displaying after applying the above styles, apply transparent background color to the root div element of the parent component
Ex: VueJS >> App.vue component
```html