Expr
[!IMPORTANT]
The repository github.com/antonmedv/expr moved to github.com/expr-lang/expr.

Expr is a Go-centric expression language designed to deliver dynamic configurations with unparalleled accuracy, safety, and speed.
Expr combines simple syntax with powerful features for ease of use:
// Allow only admins and moderators to moderate comments.
user.Group in ["admin", "moderator"] || user.Id == comment.UserId
// Determine whether the request is in the permitted time window.
request.Time - resource.Age < duration("24h")
// Ensure all tweets are less than 240 characters.
all(tweets, len(.Content) <= 240)
Features
Expr is a safe, fast, and intuitive expression evaluator optimized for the Go language.
Here are its standout features:
Safety and Isolation
- Memory-Safe: Expr is designed with a focus on safety, ensuring that programs do not access unrelated memory or introduce memory vulnerabilities.
- Side-Effect-Free: Expressions evaluated in Expr only compute outputs from their inputs, ensuring no side-effects that can change state or produce unintended results.
- Always Terminating: Expr is designed to prevent infinite loops, ensuring that every program will conclude in a reasonable amount of time.
Go Integration
- Seamless with Go: Integrate Expr into your Go projects without the need to redefine types.
Static Typing
User-Friendly
- Provides user-friendly error messages to assist with debugging and development.
Flexibility and Utility
- Rich Operators: Offers a reasonable set of basic operators for a variety of applications.
- Built-in Functions: Functions like
all
, none
, any
, one
, filter
, and map
are provided out-of-the-box.
Performance
- Optimized for Speed: Expr stands out in its performance, utilizing an optimizing compiler and a bytecode virtual machine. Check out these benchmarks for more details.
Install
go get github.com/expr-lang/expr
Documentation
Examples
Play Online
package main
import (
"fmt"
"github.com/expr-lang/expr"
)
func main() {
env := map[string]interface{}{
"greet": "Hello, %v!",
"names": []string{"world", "you"},
"sprintf": fmt.Sprintf,
}
code := `sprintf(greet, names[0])`
program, err := expr.Compile(code, expr.Env(env))
if err != nil {
panic(err)
}
output, err := expr.Run(program, env)
if err != nil {
panic(err)
}
fmt.Println(output)
}
Play Online
package main
import (
"fmt"
"github.com/expr-lang/expr"
)
type Tweet struct {
Len int
}
type Env struct {
Tweets []Tweet
}
func main() {
code := `all(Tweets, {.Len <= 240})`
program, err := expr.Compile(code, expr.Env(Env{}))
if err != nil {
panic(err)
}
env := Env{
Tweets: []Tweet{{42}, {98}, {69}},
}
output, err := expr.Run(program, env)
if err != nil {
panic(err)
}
fmt.Println(output)
}
Who uses Expr?
- Google uses Expr as one of its expression languages on the Google Cloud Platform.
- Uber uses Expr to allow customization of its Uber Eats marketplace.
- GoDaddy employs Expr for the customization of its GoDaddy Pro product.
- ByteDance incorporates Expr into its internal business rule engine.
- Aviasales utilizes Expr as a business rule engine for its flight search engine.
- Wish.com employs Expr in its decision-making rule engine for the Wish Assistant.
- Argo integrates Expr into Argo Rollouts and Argo Workflows for Kubernetes.
- OpenTelemetry integrates Expr into the OpenTelemetry Collector.
- Philips Labs employs Expr in Tabia, a tool designed to collect insights on their code bases.
- CrowdSec incorporates Expr into its security automation tool.
- CoreDNS uses Expr in CoreDNS, which is a DNS server.
- qiniu implements Expr in its trade systems.
- Junglee Games uses Expr for its in-house marketing retention tool, Project Audience.
- Faceit uses Expr to enhance customization of its eSports matchmaking algorithm.
- Chaos Mesh incorporates Expr into Chaos Mesh, a cloud-native Chaos Engineering platform.
- Visually.io employs Expr as a business rule engine for its personalization targeting algorithm.
- Akvorado utilizes Expr to classify exporters and interfaces in network flows.
- keda.sh uses Expr to allow customization of its Kubernetes-based event-driven autoscaling.
- Span Digital uses Expr in it's Knowledge Management products.
- Xiaohongshu combining yaml with Expr for dynamically policies delivery.
- Melrōse uses Expr to implement its music programming language.
- Tork integrates Expr into its workflow execution.
- Critical Moments uses Expr for it's mobile realtime conditional targeting system.
- WoodpeckerCI uses Expr for filtering workflows/steps.
Add your company too
License
MIT
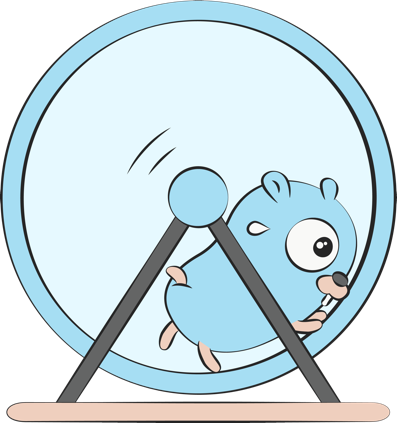