Closed xpngzhng closed 5 years ago
Did you slove this problem? I met this problem when i used pytorch0.4.1 & torchvision 0.2.1 converting resnet18
@buleZia It may be a mismatch between the caffe.proto of the caffe installed in your computer and that in Caffe directory inside this repo. Follow the advice in Caffe/ReadMe.md, replace the caffe.proto with yours and recompile to get caffe_pb2.py
PyTorch version 1.1.0 torchvision version 0.2.0
I add the following two lines after https://github.com/hahnyuan/nn_tools/blob/master/example/testify_pytorch_to_caffe_example.py#L60
then I can get perfect alexnet model conversion from pytorch to caffe.
But I failed in converting resnet18. I get the following error message:
then I checked the resnet18 prototxt, add_blob10 only apprears once:
the full resnet18 prototxt is here resnet18.txt All the element wise add operations are missing
draw the net in netscope, I see fragmented nets http://ethereon.github.io/netscope/#/editor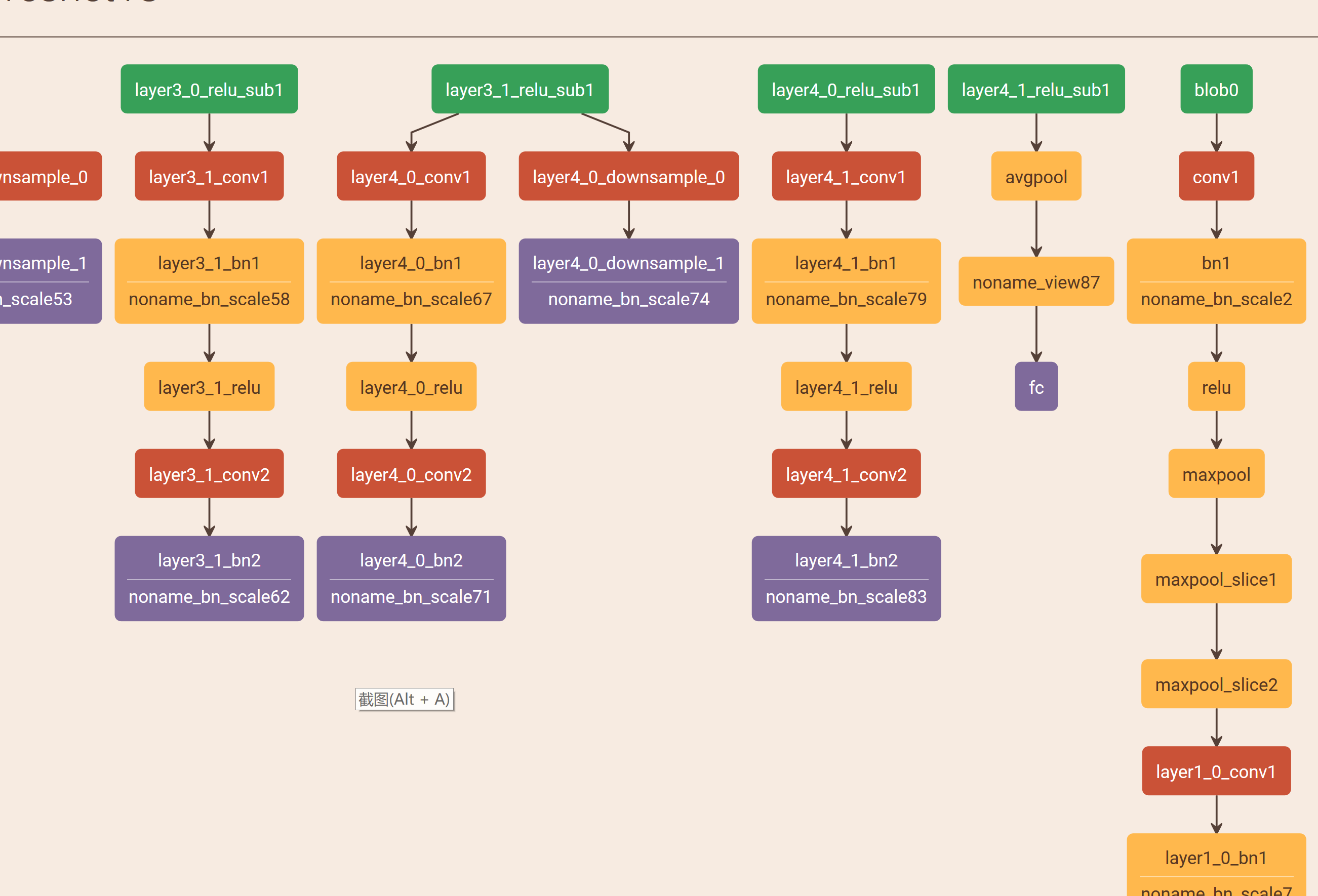
For your information, the resnet_pytorch_to_caffe.py and testify_pytorch_to_caffe_example.py are as follows, somewhat different from the original versions. I did not load the pretrained model, but save the pth model in the current directory for later testing.