Closed gydence closed 1 year ago
That last issue ended up being an issue in the drei shader. 'vNormal` isn't normalized, so this fixes the issue:
vNormal = normalize((viewMatrixInv * vec4(normalMatrix * transformedNormal.xyz, 0.0)).xyz);
@gydence Sorry, this issue is outdated, but are you able to share the final code?
Hi! I'm very new to React so apologies if this question doesn't make sense.
I'm working on a simple React app using A-Frame and I'm hoping to incorporate elements from drei (e.g. MeshRefractionMaterial). I just have a React component that has a top level
<a-scene>
and then some entities.Is there a "proper" way to mix drei components with say, a custom A-Frame JS component? I can't just instantiate it in JS:
I understandably get:
So I tried:
and then grabbing it in JS with:
But that gives me:
And even if the id worked, I'm not sure this is the correct way of doing this.
Any help is much appreciated, thank you!
EDIT:
Aha! I see that there are actually two
MeshRefractionMaterial
s in the package, one from@react-three/drei
, which is the React component, and one from@react-three/drei/materials/MeshRefractionMaterial
which is the JS material I want, just without the setup. So I can do:This gets me much closer, but now I see the mesh rendering all black and I get:
EDIT 2:
It seems that the problem was the PMREM texture. If I instead load a texture using TextureLoader (and set some of the same props that the React MeshRefractionMaterial normally does for you):
The errors go away, but the diamond renders mostly black with a sliver of my envMap colors around the edge: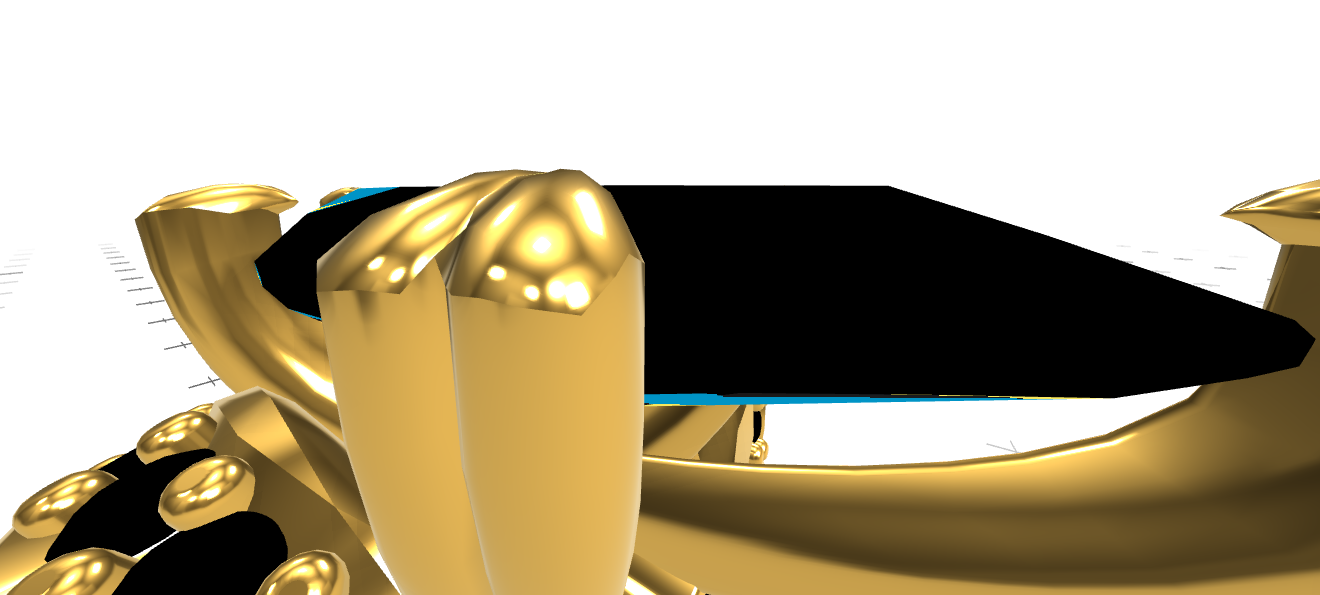