Incridea client repo
Tech Stack
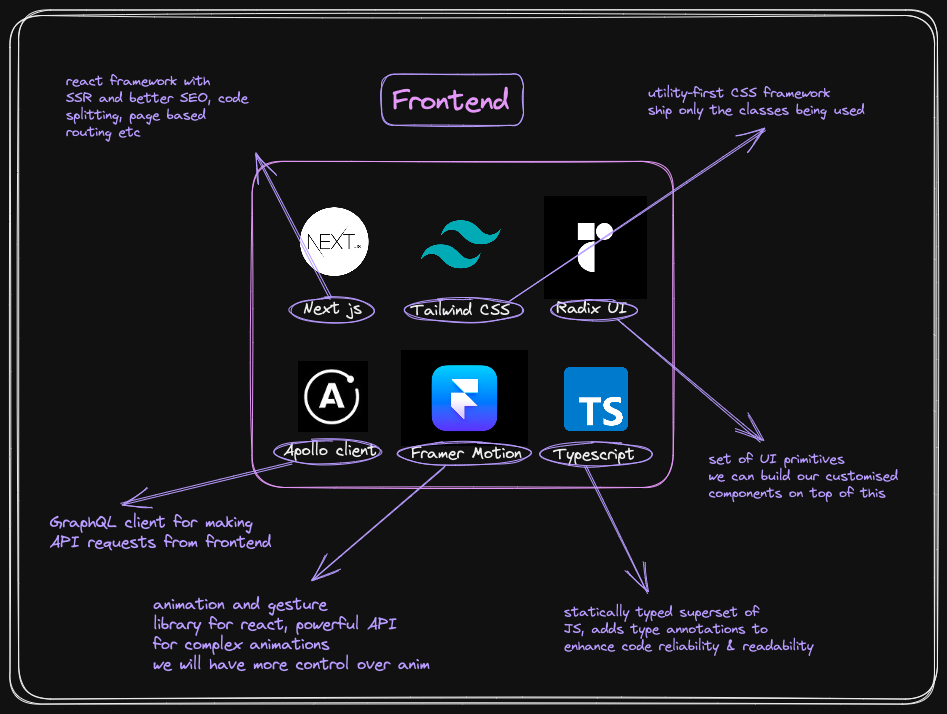
Docs
Local setup
- Clone the repository
git clone https://github.com/incridea-23/incridea-client.git
- Install all dependencies
npm i
- Run the development server:
npm run dev
Open http://localhost:3000 with your browser to see the result.
Apollo client workflow
-
Write a query or mutation under /src/graphql folder. Refer Playground for syntax.
Query Example
```graphql
query GetAllUsers {
users {
id
name
email
}
}
```
Mutation Example
```graphql
mutation SignUp($email: String!, $name: String!, $password: String!) {
signUp(data: { email: $email, name: $name, password: $password }) {
__typename
... on Error {
message
}
... on MutationSignUpSuccess {
__typename
}
}
}
```
-
Run the below command to generate the types for your queries/mutations.
npm run codegen
- Refer the below examples for queries and mutations.
Querying example
Data fetching options in Next.js
1. CSR
```typescript
import { useQuery } from "@apollo/client";
import { NextPage } from "next";
import { GetAllUsersDocument } from "../generated/generated";
const CSR: NextPage = () => {
const { data, loading, error } = useQuery(GetAllUsersDocument);
const users = data?.users;
return (
{loading &&
Loading...
}
{error &&
Error: {error.message}
}
{users?.map((user) => (
{user.name}
))}
);
};
export default CSR;
```
2. SSG
```typescript
import { NextPage } from "next";
import { GetAllUsersDocument } from "../generated/generated";
import { initializeApollo } from "../lib/apollo";
const SSG: NextPage<{
users: {
id: string;
name: string;
}[];
}> = ({ users }) => {
return (
{users.map((user) => (
{user.name}
))}
);
};
export const getStaticProps = async () => {
const apolloClient = initializeApollo();
const res = await apolloClient.query({
query: GetAllUsersDocument,
});
return {
props: {
users: res.data.users,
},
};
};
export default SSG;
```
3. SSR
Replace `getStaticProps` to `getServerSideProps`.
4. ISR
Add an invalidate option to SSG.
5. On-demand ISR
[Read about it here.](https://nextjs.org/docs/basic-features/data-fetching/incremental-static-regeneration#on-demand-revalidation)
Mutation example
Example
```typescript
import { SignUpDocument } from '@/src/generated/generated';
import { useMutation } from '@apollo/client';
signUpMutation({
variables: {
name: userInfo.name,
email: userInfo.email,
password: userInfo.password,
},
})
.then((res) => {
if (res.data?.signUp.\_\_typename === 'MutationSignUpSuccess') {
router.push('/auth/verify-email');
}
})
.catch((err) => {
return err;
});
````
Note
Refer Sign up mutation for full code.
Branching and Making PRs
- After cloning and setting up the environment, checkout to a new branch (name is related to your task, for eg: feat/user-login, fix/image-overflow)
git checkout -b <branch_name>
- Make the required changes according to your task.
//Staging changes
git add .
//Commiting changes
git commit -m <short message about task>
//Pushing changes
git push origin <branch_name>
- Make a Pull request to main branch, and wait for it to get reviewed by someone in the team. If there are review comments, make a new commit making those changes to the same branch to address those comments.
Development Notes
- Please join Incridea org on Trello from the invite link shared on Discord.
- Use the
HeadComponent
while developing a new page and provide it with suitable title and description for better SEO.
- Use semantic commit messages to keep the commit history clean.
Semantic commits
```
[optional scope]:
[optional body]
[optional footer(s)]
```
- feat – a new feature is introduced with the changes
- fix – a bug fix has occurred
- chore – changes that do not relate to a fix or feature and don't modify src or test files (for example updating dependencies)
- refactor – refactored code that neither fixes a bug nor adds a feature
- docs – updates to documentation such as a the README or other markdown files
- style – changes that do not affect the meaning of the code, likely related to code formatting such as white-space, missing semi-colons, and so on.
- test – including new or correcting previous tests
- perf – performance improvements
- ci – continuous integration related
- build – changes that affect the build system or external dependencies
- revert – reverts a previous commit